Here's an simple example. Suppose you want to display the titles of your 5 latest posts in a list. In that case, you can add the following code in to a Divi PHP Code Module:
global $wpdb;
// SQL query to fetch latest 5 posts
$query = "SELECT post_title, post_date FROM {$wpdb->posts} WHERE post_type = 'post' AND post_status = 'publish' ORDER BY post_date DESC LIMIT 5";
// Execute the query
$results = $wpdb->get_results($query);
// Display the post titles, if any, as a list
if (!empty($results)) {
echo '<ul>';
foreach ($results as $post) {
echo '<li>' . esc_html($post->post_title) . '</li>';
}
echo '</ul>';
} else {
echo 'No posts found.';
}
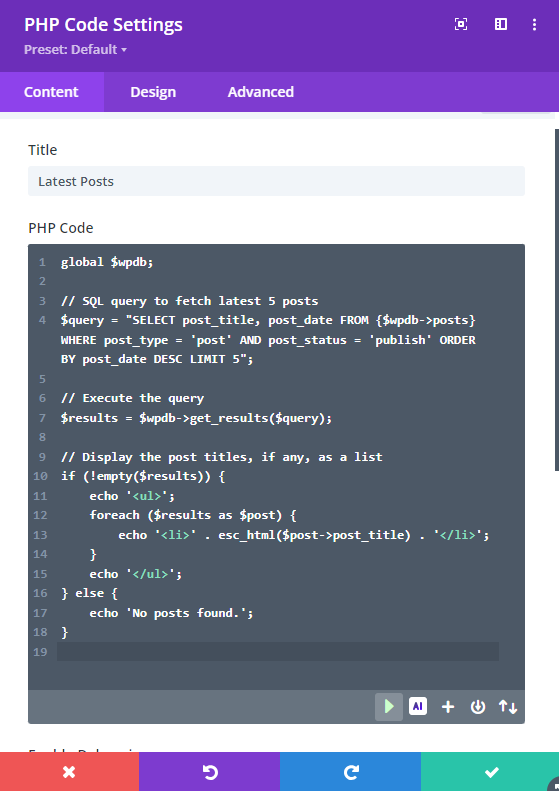
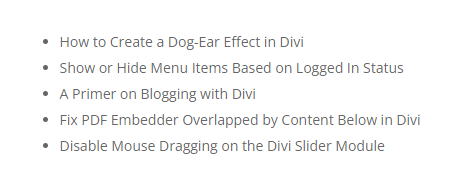
We've used the $wpdb object here as it provides a clean way to interact with the database via SQL, but you can also use PHP's raw SQL functions should you need to.
0 Comments