If you develop custom Divi modules, you may be aware of the "multiple_checkbox" field type. It lets you add settings into your Divi modules which consist of a list of checkboxes, like this:
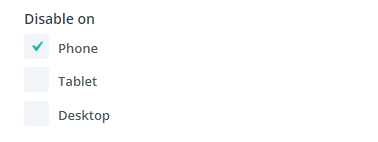
One issue you may encounter with this field type is that it doesn't handle changes to the set of checkboxes (e.g. "Phone", "Tablet" and "Desktop" in the example above) well. When the module settings are saved, the setting is stored as a list of the checkbox states, e.g. "on|off|off". That's fine as long as the list of checkboxes remains static. But suppose we wanted to change the list above to "Smartwatch", "Phone", "Desktop". Now when a module previously saved with "Phone" checked is loaded, the "on|off|off" state will cause "Smartwatch" to be checked, rather than "Phone".
One way to resolve this is to create a custom Divi module field type which resembles the "multiple_checkbox" type but stores the actual ids associated with the checked checkbox options, rather than the on / off state. Here's an example of a JSX component that will add such a field type:
// Like the built-in multiple checkboxes field, but preserves the IDs of the checkboxes in the value.
import React, { Component } from 'react';
import $ from 'jquery';
class DBC_Multiple_Checkboxes_With_IDs extends Component {
static slug = 'dbc_multiple_checkboxes_with_ids';
state = {
checkedBoxes: [],
};
constructor(props) {
super(props);
this.state = {
checkedBoxes: this.props.value ? this.props.value.split(',') : [],
};
}
_onCheckboxChange = (event) => {
const { value, checked } = event.target;
this.setState(prevState => {
if (checked) {
return { checkedBoxes: [...prevState.checkedBoxes, value] };
} else {
return { checkedBoxes: prevState.checkedBoxes.filter(box => box !== value) };
}
}, () => {
this.props._onChange(this.props.name, this.state.checkedBoxes.join(','))
});
}
render() {
let checkboxes_data = this.props.fieldDefinition.options;
const checkboxes = Object.keys(checkboxes_data).map((id, index) => {
return (
<p className="et-fb-multiple-checkbox" key={index}>
<label htmlFor={`${this.constructor.slug}-${this.props.name}-checkbox-${id}`}>
<input
type="checkbox"
id={`${this.constructor.slug}-${this.props.name}-checkbox-${id}`}
name={`${this.constructor.slug}-${this.props.name}-checkbox`}
value={id}
data-text={checkboxes_data[id]}
onChange={this._onCheckboxChange}
checked={this.state.checkedBoxes.includes(id.toString())}
/>{checkboxes_data[id]}
</label>
</p>
);
});
return (
<div className={`${this.constructor.slug}-wrap et-fb-multiple-checkboxes-wrap`}>
{checkboxes}
<input
id={`${this.constructor.slug}-${this.props.name}`}
name={this.props.name}
value={this.props.value}
type='hidden'
/>
</div>
);
}
}
export default DBC_Multiple_Checkboxes_With_IDs;
$(window).on('et_builder_api_ready', (event, API) => {
API.registerModalFields([DBC_Multiple_Checkboxes_With_IDs]);
});
Replace the DBC / dbc prefixes throughout with your own. Then compile and load the JSX component via your preferred method. I've designed it to work as a standalone component that can be manually enqueued using the 'et_fb_enqueue_assets' action. You may need to modify it slightly if using the create-divi-extension tool.
Now you can use it in your module definition like so:
'your_field_key' => array(
'label' => esc_html__( 'Multiple Checkbox Example', 'et_builder' ),
'type' => 'dbc_multiple_checkboxes_with_ids',
'options' => array(
123 => esc_html__('Item 1', 'et_builder'),
456 => esc_html__('Item 2', 'et_builder'),
),
)
Then the 'your_field_key' prop should get populated with a comma-separated list representing the IDs selected in the module setting, e.g.:
123,456
With that in place you should be able to dynamically modify the list of checkboxes displayed – as long as you keep a consistent ID for each item you can freely add, delete and reorder the items.
0 Comments