Step 1: Add PHP Code
First, we'll add some PHP code that will do the heavy lifting for us. You only need to add it once, and shouldn't need to modify it. It's main function is to scan the content of the page for Divi's video slider shortcode and add a video slide item for each of an array of videos.
class DBCDynamicVideoSlider {
public function __construct($id, array $videos) {
$this->id = $id;
$this->videos = $videos;
}
public function init() {
add_filter('the_content', array($this, 'modify_slider'));
}
function modify_slider($content) {
return preg_replace_callback(
'/(\[et_pb_video_slider [^]]*module_id="'.preg_quote($this->id, '/').'"[^]]*\])(.*?)(\[\/et_pb_video_slider\])/is',
array($this, 'add_slides'),
$content
);
}
function add_slides($match) {
$items = array();
foreach($this->videos as $url) {
$items[] = str_replace(
' src="https://www.youtube.com/watch?v=FkQuawiGWUw"',
' src="'.esc_attr($url).'"',
$match[2]
);
}
return $match[1].implode('', $items).$match[3];
}
}
Related Post: Adding PHP to the Divi Theme
Step 2: Set up the Video Slider Module
First, add a video slider module to your page. It should by default have a single video, called "New Item" configured with the URL for a video about Divi, i.e.:
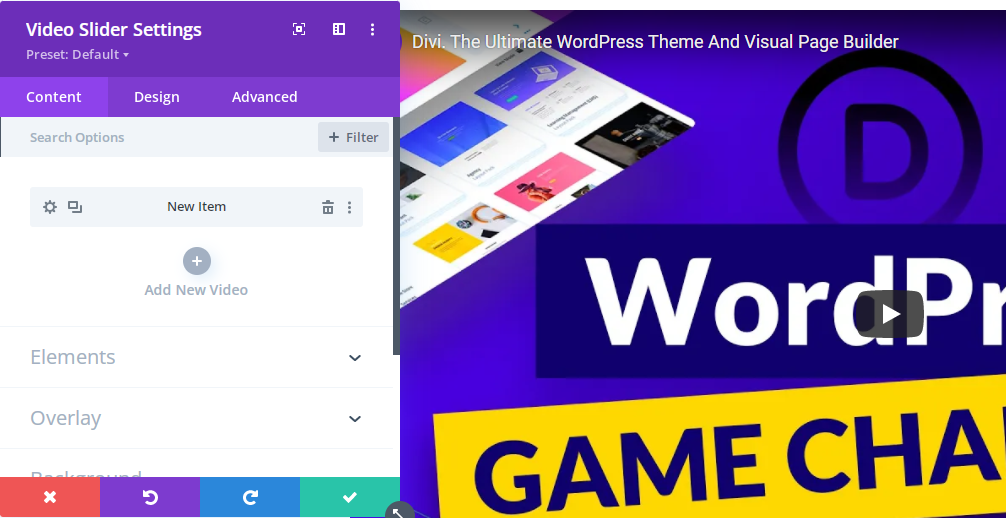
Leave that video configured as is. You can style it if you like, but leave the Divi video in place. Our code below will use the item as a template. Don't delete the "New Item" video, or add any other items.
Now give the Video Slider module a CSS ID of (for example) "dynamic-slider" at:
Video Slider Settings > Advanced > CSS ID & Classes > CSS ID
Like so:
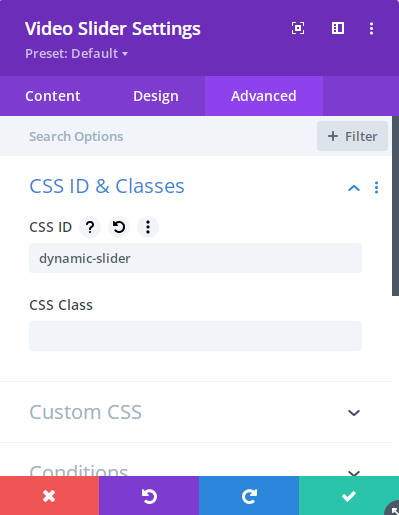
Step 3: Load the Videos via PHP
Now we need to call our earlier PHP code with the CSS ID of our slider and a list of videos to use. Here I'm just specifying the videos in a PHP array directly, but in a more realistic scenario these might be pulled in from a custom field, or retrieved from an external API.
$videos = array(
'https://www.youtube.com/watch?v=cbP2N1BQdYc', // cat video #1
'https://www.youtube.com/watch?v=lZqqMtW87hU', // cat video #2
'https://www.youtube.com/watch?v=jk0is7dZrK0', // cat video #3
'https://www.youtube.com/watch?v=uHKfrz65KSU', // cat video #4
);
(new DBCDynamicVideoSlider('dynamic-slider', $videos))->init();
Step 4: View the Result
If all is well, we should now see our videos displayed in the video slider on the front-end, e.g.:
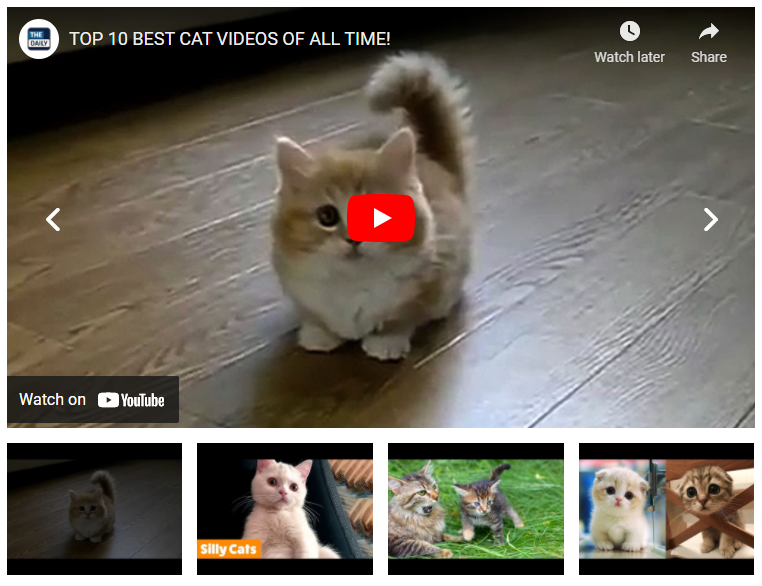
Hi Dan, Thanks for this! I actually need just one video to be shown. But this code probably won't work on the Video module. If I only add 1 video, will it just show 1 without 3 empty fields below?
Thanks, Elisabeth
Hey Elisabeth, you're right, it won't work on the Video module without modification. But in the Video slider if you just change the last block of code to just specify one video like so:
Then it should just add a single slide to the Video slider (without adding any empty fields). I haven't tested directly with this code yet, but normally when you add a single slide to the Video Slider module it just displays the same way as the Video module does – i.e. the video with no navigation arrows or thumbnails.
So I think that should do what you want. If it doesn't work the way you expect, though, give me a shout and I'll take a look for you. Thanks!