This post describes how to show, hide or toggle Divi Builder elements at the click of a button.
Reveal Elements on Click using Divi Show / Hide Module
Divi Show / Hide Button Module is a plugin I designed to make it easier to implement some of the effects described later in this post – without the need to mess around with jQuery code.
It is a modified version of the built-in Divi Button module. Rather than linking to another page, it lets you show, hide and toggle the visibility of elements on the same page. It can be styled the same way as the standard button module.
Check out the Divi Show / Hide Button Module here.
Reveal Elements on Click Using CSS / JQuery
Rob over at Divi Notes has a nice post on how to reveal a Divi section, row or module when a button is clicked. I've made what I think are a couple of improvements, which are shared below. The changes include:
- Preventing the hidden component from being briefly displayed when the page loads
- Support for multiple hidden sections / reveal buttons
- No hiding of the component in the visual builder for easier editing
Here are the steps to making a Divi Builder element (module, etc) displayed on the click of a button (and re-hidden if the button is clicked again).
Step 1: Add the "reveal" button and give it a class
Add a Button Module to your page that you want to use to show / reveal the target element.
Then set
Button Settings > Advanced > CSS ID & Classes > CSS Class
to
rv_button_1 rv_button_closed
like so:
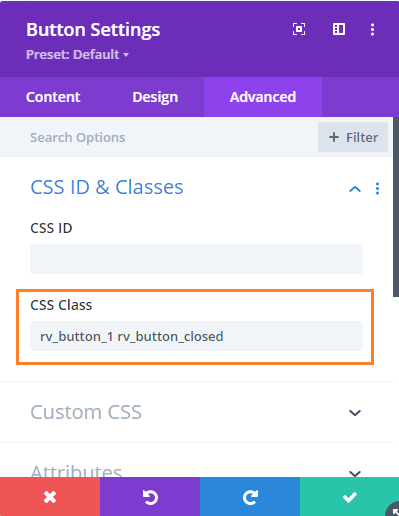
Step 2: Add the hidden element and give it a class
Now add the element you want to show / hide. This can be a section, row or module. Go to the settings for the element, e.g.
{Element} Settings > Advanced > CSS ID & Classes > CSS Class
And add the following to the CSS Class field:
rv_element rv_element_1
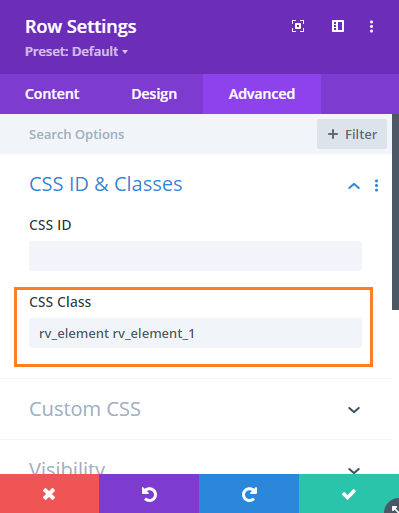
Step 3: Add the following CSS / JavaScript code to your site
<style>
body:not(.et-fb) .rv_element { display: none; }
.et_pb_button.rv_button_opened:after { content:"\32"; }
.et_pb_button.rv_button_closed:after { content:"\33"; }
</style>
Related Post: Adding CSS to the Divi Theme
<script>
jQuery(function($){
var revealButtons = {
'.rv_button_1': '.rv_element_1'
};
$.each(revealButtons, function(revealButton, revealElement) {
$(revealButton).click(function(e){
e.preventDefault();
$(revealElement).slideToggle();
$(revealButton).toggleClass('rv_button_opened rv_button_closed');
});
});
});
</script>
Related Post: Adding JavaScript / jQuery to Divi.
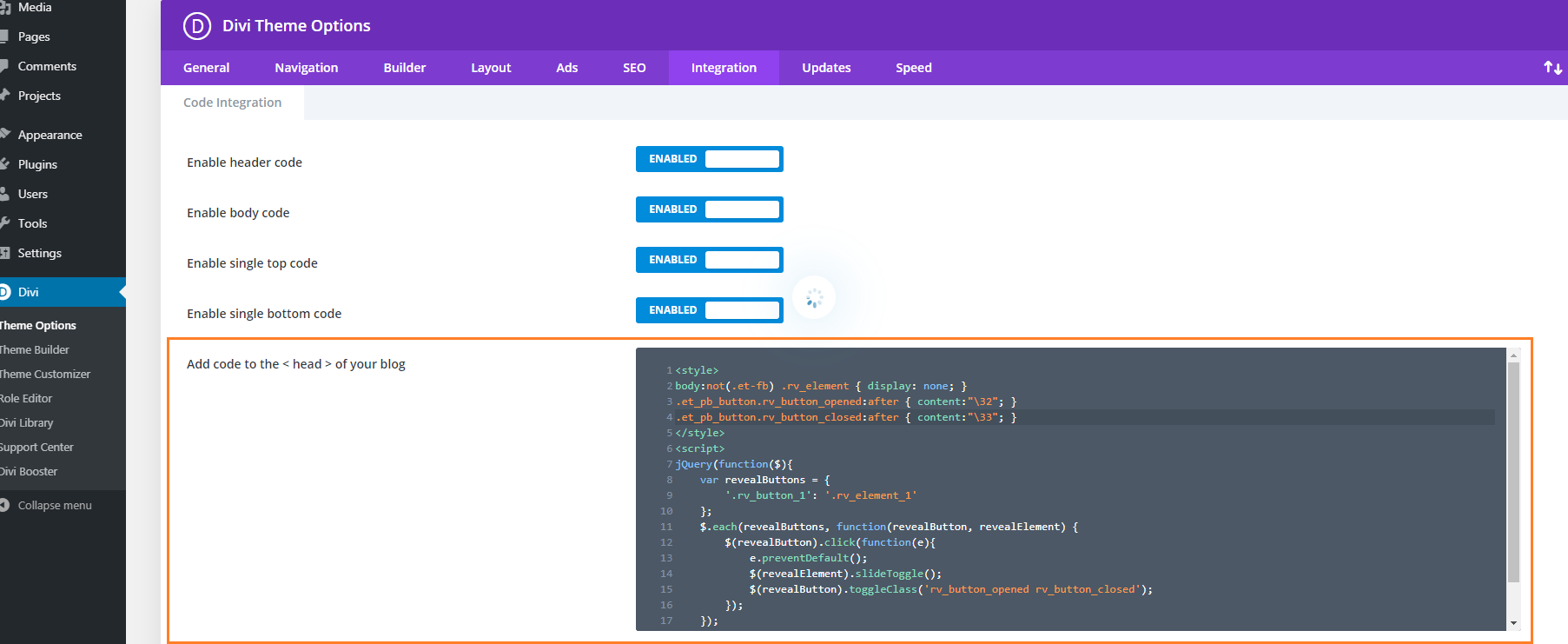
Step 4: Publish and view the page
If all has gone well, the element should be hidden on the page. Clicking on the reveal button should reveal the element, and clicking a second time should hide it again.
If you have any trouble getting it to work, let me know in the comments or via the contact form.
It should look something like this:
Before:

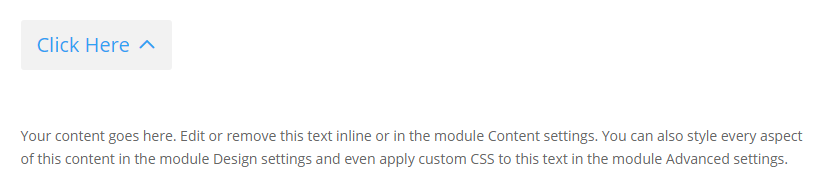
Advanced: Adding more reveal buttons
If you want to add further reveal buttons (which reveal their own elements), you can do it like so:
- Give each new reveal button its own CSS class, for example "rv_button_2" for the second reveal button, "rv_button_3" for the third and so on.
- Give each new hidden element its own CSS class, for example "rv_element rv_element_2" for the second element, "rv_element rv_element_3" for the third, and so on.
- Modify the JavaScript code to include these new classes, e.g.
<script>
jQuery(function($){
var revealButtons = {
'.rv_button_1': '.rv_element_1',
'.rv_button_2': '.rv_element_2',
'.rv_button_3': '.rv_element_3'
};
$.each(revealButtons, function(revealButton, revealElement) {
$(revealButton).click(function(e){
e.preventDefault();
$(revealElement).slideToggle();
$(revealButton).toggleClass('rv_button_opened rv_button_closed');
});
});
});
</script>
Advanced 2: Showing and hiding elements on click
If you want to do something more than just revealing an element when the reveal button is clicked, here's an extended version of the code above, which adds the ability to show, hide and toggle multiple elements from the same button. This lets you do things like hide an element when revealing another, or replacing the reveal button with content (by hiding the reveal button once clicked).
<style>
body:not(.et-fb) .show-on-click,
body:not(.et-fb) .toggle-on-click {
display: none;
}
.et_pb_button.rv_button_opened:after { content:"\32"; }
.et_pb_button.rv_button_closed:after { content:"\33"; }
</style>
<script>
jQuery(function($){
var buttons = {
'.rv_button_1': {
'toggle': '.toggle-on-click',
'hide' : '.hide-on-click',
'show' : '.show-on-click'
}
};
$.each(buttons, function(button, elements) {
$(button).click(function(e){
e.preventDefault();
$(elements.toggle).slideToggle();
$(elements.show).slideDown();
$(elements.hide).slideUp();
$(button).toggleClass('rv_button_opened rv_button_closed');
});
});
});
</script>
The biggest change is that the buttons array now defines "toggle", "hide" and "show" values for the button(s).
- "toggle" takes a comma-separated list of CSS selectors which will be alternated between hidden and visible with each click of the reveal button. This is the default behavior of the earlier code examples.
- "hide" takes a comma-separated list of CSS selectors which will be hidden when the button is clicked. Subsequent clicks won't affect their visibility.
- "show" takes a comma-separated list of CSS selectors which will be displayed when the button is clicked. Subsequent clicks won't affect their visibility. It makes sense to hide such elements initially using CSS, as done for the ".show-on-click" class in the style block above.
Setup the button as per step 1, and give each element you want to be affected by the button the appropriate class (in the case of the example code this would be either "toggle-on-click", "hide-on-click" or "show-on-click").
Now you should see an effect similar to this (left column is set to toggle, center to show, and right to hide):
Initial state:
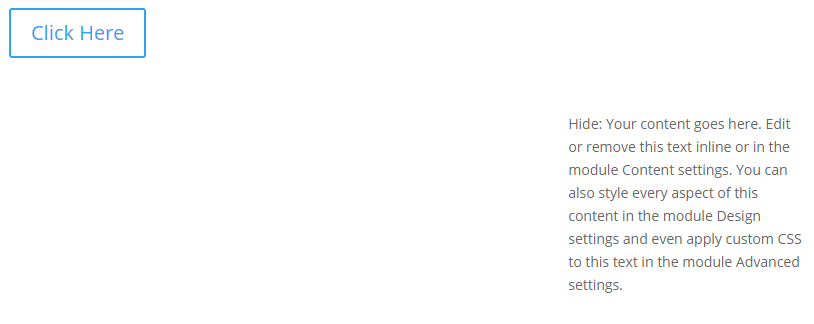
After first click:
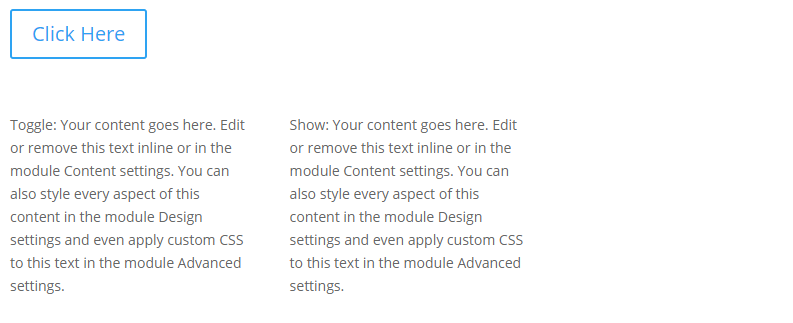
After second click:
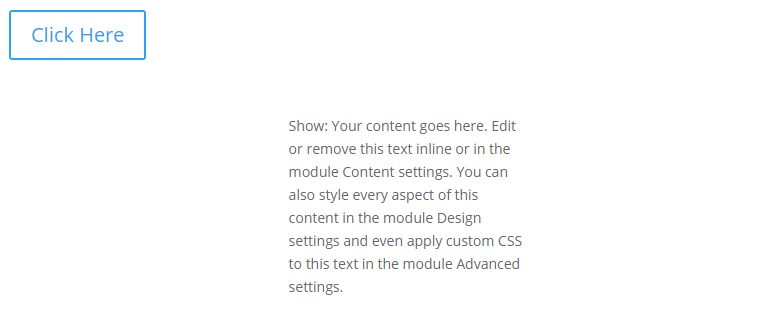
Advanced 3: Changing the Open / Close Icons
you can change the open / close icon from the default up / down arrow by changing these lines of CSS:
.et_pb_button.rv_button_opened:after { content:"32"; }
.et_pb_button.rv_button_closed:after { content:"33"; }
Here '32' is the code for the up arrow icon and '33' is the code for the down arrow icon. You can easily change this to another icon from this list:
https://www.elegantthemes.com/blog/resources/elegant-icon-font
Go to the "Complete Set and Unicode Reference Guide" section and then locate your desired icon(s). Take the part of the icon's code as shown here:
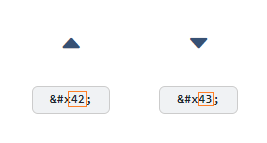
.et_pb_button.rv_button_opened:after { content:"42"; }
.et_pb_button.rv_button_closed:after { content:"43"; }
Advanced 4: Hiding Other "Tabs" Initially
If you have several reveal buttons and want to create a "tabbed" effect, you may wish to simulate a click on the first reveal button to hide the content of the other "tabs". Here's two ways to do it:
1) Hide the other tab content elements initially via CSS, such as:
.rv_element_2,.rv_element_3,.rv_element_4 {
display: none;
}
Note that you may need to change these class names to match your setup.
You can add this into your child theme style.css file or the "Divi > Theme Options > General > Custom CSS" box.
2) Trigger a click event on the button using jQuery. You should be able to do so by adding this after the jQuery code you've already added above:
jQuery(function($){
$('.rv_button_1').click();
});
Where "rv_button_1" is the class assigned to your first "tab" button.
The two options should achieve pretty much the same result, but there are some slight differences. The first option will apply immediately, while the second option only applies once the page is fully loaded, which might lead to the rows being briefly visible while the page is loading. The second option, though, more accurately simulates a button click which might be useful if you ever attach more complex behavior to the button.
Related post: Creating Tabs using the Divi Show / Hide Button Module
Advanced 5: Sliding the Revealed Element Left / Right
In the examples above, the element being revealed slides up / down as it is revealed / hidden. There isn't an equivalent way in jQuery to instead slide it left / right. However, we can achieve a left / right slide effect by additionally making use of the jQuery UI library.
First, install the jQuery UI library on your page / site following the instructions on the jQuery UI site. This will likely involve adding a script tag such as this to your site:
<script src="https://cdnjs.cloudflare.com/ajax/libs/jqueryui/1.13.2/jquery-ui.min.js" integrity="sha512-57oZ/vW8ANMjR/KQ6Be9v/+/h6bq9/l3f0Oc7vn6qMqyhvPd1cvKBRWWpzu0QoneImqr2SkmO4MSqU+RpHom3Q==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Important: To get jQuery UI to work correctly, you may also need to disable Divi's jQuery Deferral option at:
Divi > Theme Options > General > Performance > Defer jQuery And jQuery Migrate
This in the original example (given at the top of this post), replace the line that reads:
$(revealElement).slideToggle();
With this (to slide right):
$(revealElement).toggle("slide", { direction: "left" }, 1000);
Or this (to slide right):
$(revealElement).toggle("slide", { direction: "right" }, 1000);
It is worth noting that loading jQuery UI and disabling Divi's jQuery Deferral may impact the page / site speed to some degree, so be sure to check that you are happy with the resulting site performance when implementing this.
Advanced 6: Deactivating Previously Active Buttons
In the above examples, a button which has been clicked becomes "active" and can be styled using the "rv_button_opened" class added to it. When the button is clicked again the class is removed, removing the active styling. However, buttons added using the instructions above act independently: activating one button doesn't "deactivate" the others. If you would actually like a newly active button to deactivate others (so you only have one button active at a time), then one way to handle this situation is to modify the code such that when the button is clicked, it removes the rv_button_opened class from the other buttons. Adding a line like this should do it:
$('.et_pb_button.rv_button_opened').not(revealButton).removeClass('rv_button_opened').addClass('rv_button_closed');
This is how it would look added to the code in the "Advanced: Adding more reveal buttons" section:
jQuery(function($){
var revealButtons = {
'.rv_button_1': '.rv_element_1',
'.rv_button_2': '.rv_element_2',
'.rv_button_3': '.rv_element_3'
};
$.each(revealButtons, function(revealButton, revealElement) {
$(revealButton).click(function(e){
e.preventDefault();
$(revealElement).slideToggle();
$(revealButton).toggleClass('rv_button_opened rv_button_closed');
$('.et_pb_button.rv_button_opened').not(revealButton).removeClass('rv_button_opened').addClass('rv_button_closed');
});
});
});
Note that if you use it with the code in "Advanced 2: Showing and hiding elements on click" you'd instead need to use:
$('.et_pb_button.rv_button_opened').not(button).removeClass('rv_button_opened').addClass('rv_button_closed');
(as it uses "button" as the variable name, instead of "revealButton").
For users of the Divi Show / Hide Button module, the grouping option can be used to make a set of buttons act as a group with only one button active at a time. The currently active button can then be styled using the active button styling options.
Advanced 7: Only Show the Button on Mobile
To only show the button on mobile:
1. Click on the "disable" option in the button module's menu:
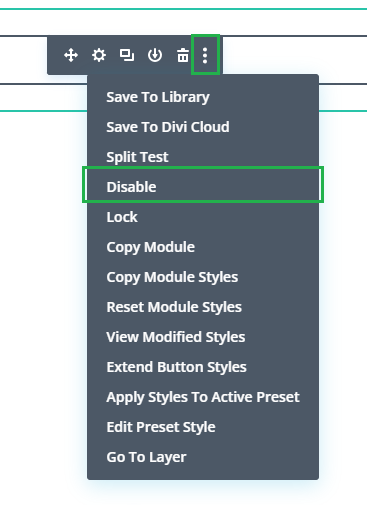
2. Click on the "tablet" and "desktop" icons, to disable the button module on those devices. This will make the button show on mobile only.
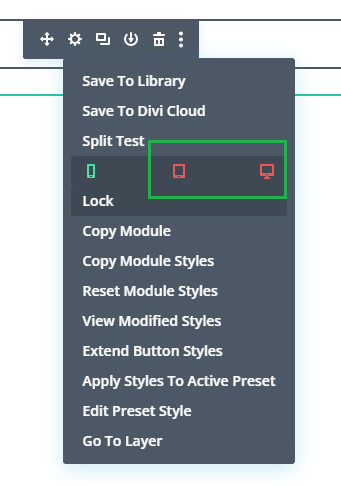
3. Wrap the CSS code from the tutorial in a "media query" which will make the CSS code only apply on mobile (leaving the filters visible on tablet / desktop). For example, if you are using this CSS from above:
<style>
body:not(.et-fb) .rv_element { display: none; }
.et_pb_button.rv_button_opened:after { content:"\32"; }
.et_pb_button.rv_button_closed:after { content:"\33"; }
</style>
You'd change it to this:
<style>
@media only screen and (max-width: 980px) {
body:not(.et-fb) .rv_element { display: none; }
.et_pb_button.rv_button_opened:after { content:"\32"; }
.et_pb_button.rv_button_closed:after { content:"\33"; }
}
</style>
This would benefit from some pictures or an example. As someone with ADHD, even though i know my answer is probably hidden somewhere in this article. The wall of text is super off-putting :(
Hi Adhd lady, I've added some screenshots. I hope they help you out, but let me know if anything remains unclear. Thanks!
This is awesome and works perfectly! Is there a way I can have the hidden section replace the section with the button entirely? I'm essentially trying to have tutorial text show up and a button that says "Join now". Once someone clicks the button, the section with the button is replaced by the hidden content. Is there a way to do this? Thank you!
Hi Meg, I've just added a new section "Advanced 2: Showing and hiding elements on click" which gives a way to hide elements when the button is clicked. If you add the "hide-on-click" class to the button, that should cause it to be hidden when the main section is revealed. Hope it helps, but let me know if not!
Hi Dan,
thanks for the solution, it is working great.
I am trying to do make one tweak and am not sure if it is possible? I would ideally like when you click on a button it to hide any previously revealed other sections. Is this achievable?
Many thanks,
Paul
Hi Paul, I've just added a section to the end of the post which gives a way to do this. Hope it is clear, but give me a shout if you can't get it to work. Thanks!
Thanks for sharing this.
Is there a way to have the button change text whether the hidden section is closed or open?
For example, let's say button has "See more" on it when the hidden section is closed. When it is clicked and the section is revealed, it no longer makes sense for "See more" to be on the button". It would be more logical to have "See less" (as an example).
Do you know of a relatively simple way to achieve this?
I think I figured it out. I would just have "See" on the button, and then use " More" and " Less" in the :after content. Should do the trick.
Okay, great! Yeah I think that should work (I'm sure I've used that trick on buttons before), but let me know if you need any help getting it going. Thanks!
Hi, Jonathan. what would the code be, for this functionality? My button would say 'Show Advisors' and 'Hide Advisors'.
Thanks! and Thank You, Dan, for the great article and info!
Hi Brian, I think what Jonathan is doing is, in his case, setting the button text to "See", and then using the following CSS to append the word "Less" or "More" after the button text like so:
So to modify this for your case, you could set the button text to "Advisors" and then use this CSS to place the correct text *before* it:
I haven't had a chance to test this yet, so if it doesn't work out or needs some extra styling, let me know (with a link to your page, if possible). Cheers!
Hi Dan,
Thanks for this bit of code. It works.. If I only wanted to do three buttons. I want to add 3 more buttons onto my page, and I've checked each bit of code to make sure this works, but only first three are working. And only two are working correctly.
I've set each row I want to load correctly in consecutive order. But my button#3 is loading my button #6 content… I'm thinking I may just have too much content on my page? Would you be able to check it out?
Ah. Nevermind! I just looked at it one more time, and I missed changing the button #s in the javascript. This works perfectly! Thank you!!
Great! I’m glad you figured it out, Sannah. Thanks for letting me know :)
Hi,
can i make that work with a menu as well? i would like to use a menu instead of the button module. sadly if i add the css to the menu item via the optional CSS fields for menus it does not work.
Hey Stefan, are you able to share a link to an example page, pointing out the menu item you want to link / the element you want to reveal? I think a few changes might be needed to both the CSS and jQuery code to make this work, but this will likely depend a bit on the setup of your menu, etc. If you're able to send through that link (or describe in more details your setup – e.g. are you using a menu module or default Divi menu, is this a submenu item, etc) I'll try to come up with a solution that works for you. Cheers!
hey Dan, thanks for the code. works fine!
here's my question: I tried to apply the class rv_button to a text module. Works, too, but: if I activate the button a 3 is added to the text in it…
Is there a way to avoid this?
Hey Julian, were you able to solve this? I took a look at the site linked in your comment and can see the text module reveal working without the "3" appearing. If you're still having problems with it, let me know. Thanks!
Hello, Dan! Thanks for posting this. I've been working on adding a custom button or call to action module which will expand a large search box when clicked on. The solution works great, however, I'm seeing a bit of a jump when the button is clicked, e.g. the search box code module jumps a bit to the top right under the button before it stabilizes. I've tried adding the CSS and jQuery code to the overall page header in Divi but it didn't work. Do you have an idea of how I can avoid that jump? I also confirmed there aren't any modified styles in the search code module either.
Thanks much, for any insight you might provide!
Hey Kory, is there any chance you're able to send me a link to an example page showing the problem so that I can take a look at how you have it set up? If not, are you able to do capture video of the effect? That should help me replicate / debug the issue. Thanks!
OK, made some mods and I think I have it working now.
Okay, great. Thanks for letting me know, Maurice. I took a look and I can see it working as you describe, but give me a shout if you have any further problems with it. Cheers!
Something isn't working here. It opens then immediately closes every time. Very strange.
I have put this line in and taken it out – no change:
#reveal { display: none; }
I actually want it to come in open and close after. But it just won't work. Ideas?
Hi Maurice,
Did you figure this one out?
I seem to have the same problem…
Thanks!
Marthe
Hi Marthe (and Maurice), I've seen this type of behavior when the button has been configured to both show and toggle the target section. In this case, when the button is clicked the section is first shown, making it visible, and straight after toggled, hiding it again (since toggling makes a visible element hidden, and vice-versa). A similar thing can happen if a section is configured to be both shown and hidden, though that's a more obvious mistake to spot. Only applying one operation to the element, e.g. show or toggle, should solve it if this is the issue for you.
If that doesn't help, perhaps you can send through a link to the page you're working on, either here or privately via the contact form, so that I can take a look for you?
Thanks Dan, I was wondering would there be any issues if I wanted to use this multiple times on my site with different hidden elements. I don't really see why there would be but doesn't hurt to ask!
Hey Tanner, if you're using it on different pages then there should be no problem at all. You can just include the CSS / JS code globally and then on any page you want to use the code, add the "rv_button" class to your "reveal button" and the "reveal" id to the module / row / section you wish to reveal.
If you want to have multiple reveal buttons / sections on a single page, you could duplicate the code and replace all the mentions of the "rv_button" class and "reveal" id with, e.g., "rv_button_2" and "reveal_2".
Hi Dan – thanks for this code. I'm attempting to add multiple reveal secants on a single page. I've tried changing code for the subsequent areas to _2 and so forth, but it's not working. Additionally, I'm seeing the "2" and "3" numbers over my images. Any help would be greatly appreciated. Thanks.
It looks like the issue is that while you've updated the code correctly, you haven't yet updated the class in the reveal button (blurb, in your case) module settings. This should be updated to match what you have in the code ("rv_button_2", etc). Likewise, you need to add the new IDs you've created for the reveal element ("reveal_2") in the CSS Class settings for the corresponding element (row, in your case). The stray "2" and "3" you're seeing in the page is a result of using the code on a blurb module instead of a button module. You can fix this by deleting the two rows of CSS that read:
Hopefully that sorts it out for you. If not, I've updated the post with a some changes to the code and further details to make it (hopefully) easier to achieve multiple reveal buttons. It should also sort out the issue with the stray numbers. Note that this would be a replacement for the code, etc, that you've already applied.
I hope that helps, but please let me know if not.
I've given this a try, and it's doing the opposite—the module I want to be hidden is visible upon page load. Clicking the button actually hides the module instead of reveals it. Any ideas?
Hi Kristin, there are a couple of things stopping it from working at the moment.
First is that the CSS code isn't being added to the page. Make sure the first block of code is added to your site, and clear any caches on your site after doing so. Depending on where you add it, you may or may not need the "style" tags. This first line of CSS (the one starting "#reveal") is the one responsible for hiding the module initially.
Second, I can see that you've set "reveal" as the ID of both the module and the section containing it. You'll need to remove the ID from one of these elements. Remove it from the section if it is the module you want to reveal, and remove it from the module if it is the whole section (padding and all) that you want to reveal. The feature won't work with both IDs present.
I hope that helps!
Thanks, Dan! We're up and running now. For some reason, the CSS code didn't take the first time around. A good lesson to double check that edits save first and foremost!
Good catch on the double reveal ID. Thanks again!
Great! I'm glad you're figured it out, Kristin, and that that's all it was. I don't want to think about how many hours I've spend debugging issues only to realise I never saved the changes… :)